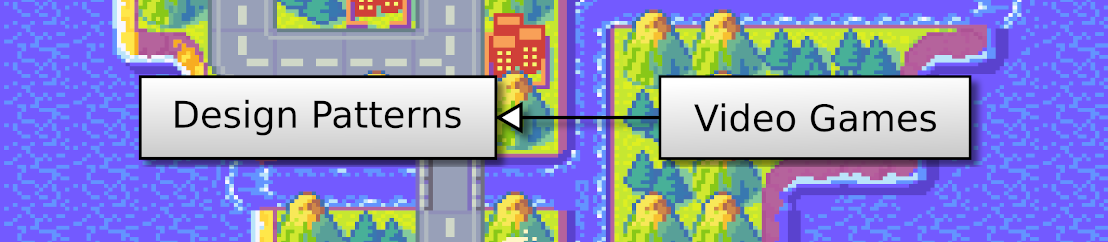
In this series, I propose to discover the Facade Pattern. In short, this pattern defines an interface that allows a reduced and simplified use of a set of features. To illustrate, we design a facade of the AWT graphics library for a 2D tile game.
Let's start with the simplest facade with a unique createWindow()
method that allows the user to create a new window:
Creating a window with this facade is very simple: we instantiate an implementation of the facade (l.1), and call the createWindow()
method (l.2):
GUIFacade gui = new AWTGUIFacade();
gui.createWindow("AWT GUI Facade");
In this example, I propose to implement a window creation with the AWT library: other implementations are possible without changing the GUIFacade
interface and its use. We define an AWTWindow
class that implements java.awt.Frame
to handle our window:
The createWindow()
method of the AWTGUIFacade
class instantiates an AWTWindow
(l .4), initializes it with a title (l.5), places it in the center of the screen (l.6) and makes it visible (l. 7):
public class AWTGUIFacade implements GUIFacade {
@Override
public void createWindow(String title) {
AWTWindow window = new AWTWindow();
window.init(title);
window.setLocationRelativeTo(null);
window.setVisible(true);
}
}
The AWTWindow
class has an init()
method that initializes the window with a given title (l.3) and a size of 640 by 480 pixels (l.4). It also prohibits resizing (l.5), and ensures that we destroy the window when closed (l.6-11):
public class AWTWindow extends Frame {
public void init(String title) {
setTitle(title);
setSize(640, 480);
setResizable(false);
addWindowListener(new WindowAdapter() {
@Override
public void windowClosing(WindowEvent we) {
dispose();
}
});
}
}
The creation of the window is split between the createWindow()
method of the AWTGUIFacade
class and the init()
method of the AWTWindow
class. The main motivation here is to separate dynamic operations from static ones. For instance, the position of the window can change, but not the title or the size of the window. There are many other possibilities depending on the possible extensions of the facade.
With this facade, a user can easily create a window for a specific use, without having to know all the methods of a graphics library. Moreover, apart from the instantiation of a layout of the facade (i.e., new AWTGUIFacade()
), all the following code no longer depends on the graphics library (the gui
variable in the first example code is of type GUIFacade
). It is easy to switch from one implementation to another, and therefore from one graphics library to another. This property is very interesting when you want to cross-platform, or if the first choice of graphics library was not good. In any case, we can switch from one library to another without having to rewrite the entire application!
This article presents the basics of the facade pattern. In the following posts, things will become more complex, and you will see what can be achieved from a pattern whose principle seems so simple!
The code of this post can be downloaded here:
To compile: javac com/learngameprog/awtfacade01/Main.java
To run: java com.learngameprog.awtfacade01.Main