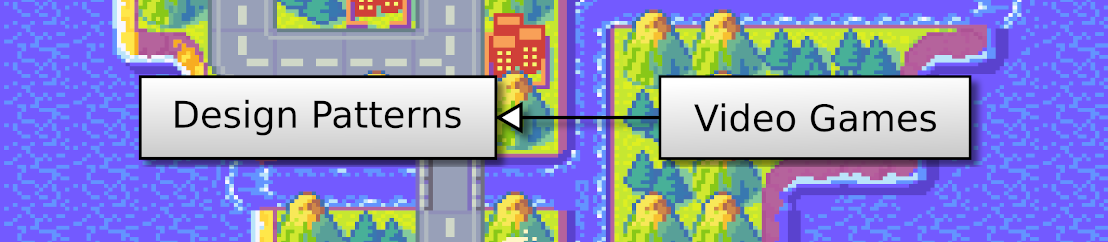
In this series, I propose to discover Python and design patterns. The Python language is today one of the best language to discover programming. At the same time, I will also introduce design patterns, a collection of programming recipes that give many good ideas when creating programs. In this first post, we see the Spyder IDE and the print() function.
This post is part of the Discover Python and Patterns series
Before starting anything we need a development environment. There are many possibilities with Python, I propose here one of the most popular: Anaconda with Spyder. Anaconda is a tool that contains Python with many libraries and applications. The default package has a lot of them, including Spyder. Later, it is easy to add/remove/upgrade these libraries and applications. Spyder is an IDE dedicated to Python, its use is simple and easy.
To get Anaconda, download the Python 3 distribution here. Run the installer, and be patient... Installation takes a while. At the end of the installation, you can check the boxes if this anaconda will be the main Python env you will use. Otherwise, don't touch anything, you can easily run programs from Spyder without these options. Then, if you are using Windows, search "Spyder" in the start menu, and you should see something like this:
The left part is the current Python program:
# -*- coding: utf-8 -*-
"""
Spyder Editor
This is a temporary script
"""
These lines are only comments, they don't do anything if you run it. The first line starting with a '#' is a single line comment, and the following ones are multi-lines comments, starting and ending with a triple double quote.
The upper right part shows some help - there is other tabs we'll see later.
The lower right part shows the console - this is where messages are printed.
Let's create now a new program from the menu File / New File... A new tab appears in the top left part, with already some content: this is also comments, they will not lead to any action. Type print("You win !") right after, and you should get something like:
# -*- coding: utf-8 -*-
"""
Created on Wed Oct 2 21:49:54 2019
@author: phylyp
"""
print("You win !")
Save it from the menu File / Save, for instance with the file name "win.py". Your interface should look like the following one:
This first program is a really easy game: nothing to do you always win :)
To run this program, click the play icon in the top bar:
You should see the following dialog. Check "Remove all variables before execution", it'll prevent unexpected errors later:
Then, in the console in the bottom right part, you should see the following new lines:
In [1]: runfile('C:/dev/win.py', wdir='C:/dev')
You win !
In [2]:
Your Spyder interface should look like this one:
The first line In [1]: runfile('C:/dev/win.py', wdir='C:/dev')
is a command Spyder typed itself to run the python program "C:\dev\win.py" in the directory "C:\dev" (Spyder uses slash '/' instead of '\', it works the same). Do not worry about this line.
The second line You win !
is the result of our command print("You win !")
. This command is a function call. You can recognize a function call thanks to a name followed by an open parenthesis. For instance, the following command:
print()
calls the print function.
Inside the parenthesis, we can add items called arguments. In our example, we have a single argument "You win !". The double quotes mean that this is string content, that should not be interpreted by Python. Otherwise, if you type print(You win !), the interpreter will try to execute You win !, but it has no meaning in Python.
Functions can also be called with several arguments using commas, for instance with the print function we could type:
print("You","Win","!")
It actually print the same message, since arguments of the print function are separated by spaces. For instance, if you run:
print("Wo","rd")
It will print (a space is printed between "Wo" and "rd"):
Wo rd
Python, as for all programming languages, has strict syntax rules. If you make mistakes in your program, it will be impossible to run it. For instance, if you forget the last parenthesis in the print() line:
print("You win !"
Spyder will show you that there is an error in this line with an exclamation mark left to the line. If you leave your cursor on this icon, you'll get some help:
If you run this line, you will see a long error message in the console (bottom right part of Spyder):
It tells you that there is a syntax error in your program (here "C:\dev\win.py") at line 8. The messages before this line show the execution stack, this is the history of all function calls that led to this result.
To run the program again, just add the missing parenthesis.
This first post is not very exciting, but basics have to be presented in order to better present the following topics. In the next post, we'll see first interactions with the player.