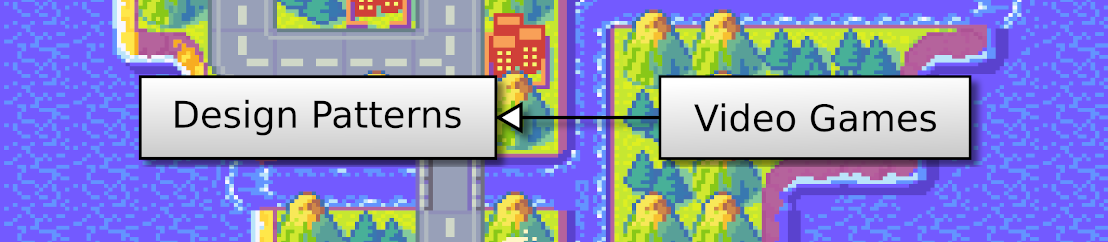
In the first post, we saw how to install an IDE and how to display a message; it is time to add some interaction with the player.
This post is part of the Discover Python and Patterns series
Create a new python program and type the following line:
input("Press Enter to continue...")
If you run the program, you get the following result in spyder:
In the spyder console, we find the same line starting with "In [1]:", and the message "Press Enter to continue...":
In [1]: runfile('C:/dev/press_enter.py', wdir='C:/dev')
Press Enter to continue...
There is no line starting with "In [2]:" as before: it means that the console is waiting for user input. Click on the console window to get the focus, and then press Enter:
Now the program is over, and the line starting with "In [2]:" is visible:
In [1]: runfile('C:/dev/press_enter.py', wdir='C:/dev')
Press Enter to continue...
In [2]:
As for the print()
function, the input()
function can have one argument: the message to display. It also has a return value: the message typed by the user. If you run the following program:
name = input("Enter your name: ")
print("Your name is",name)
and if you type your name in the Spyder console, then you get a result like:
In [1]: runfile('C:/dev/enter_name.py', wdir='C:/dev')
Enter your name: phylyp
Your name is phylyp
In [2]:
We store the return value of the input()
function in the variable name
. Then, we print the message "Your name is" followed by the content of the variable name
(with one space in between).
Note that, if we type this:
print("Your name is","name")
We get the following result:
Your name is name
Since quotes surround name
, Python does not analyze it and just copy it. If we remove the quotes (as before), we see the content of the variable name
.
There are many ways to combine strings, like the one with the arguments of the print()
function. We can also use the following syntax to create the same result:
print("Your name is {}".format(name))
With this syntax, each opening/closing braces "{}" is replaced by the value of the arguments in the following format()
function. Note well the dot .
between the message and the format()
function: I'll explain in another post what it means.
The advantage of this syntax is that spaces are not mandatory between values. For instance, if we type:
print("Your name is '{}'".format(name))
We get the following result:
Your name is 'phylyp'
With print()
arguments:
print("Your name is '",name,"'")
We get:
Your name is ' phylyp '
You can notice the spaces that surround the name.
We use the variable name
to store what the user typed. We can also use variables to store other content; for instance, we can store the string combination in a variable before printing it:
message = "Your name is '{}'".format(name)
print(message)
It does not change the final result (we print the same message), but it better shows how Python works internally. Even without the message
variable, Python first computes the string combination in a variable and then calls the print()
function with the content of this variable.
For now, remember this: functions don't trigger the computation/creation of their arguments. Python first computes function arguments and then calls the function. If there is an error during the computation of an argument, Python never calls the function.
You can also run Python programs in a system console. Open the run options dialog using the menu Run / Configuration per file... Then, select Execute in an external system terminal in the console section:
If you run the program, a system console pops up:
You can type a name as before, but once you hit the Enter key, the system console disappears. If you want to see the message "Your name...", add a third line to your program that waits for a key:
name = input("Enter your name: ")
print("Your name is",name)
input("Press Enter to continue...")
This post shows how to ask a value to the user, but the result is always the same. In the next post, I show how to branch; in other words, how to change the behavior of the program depending on cases.