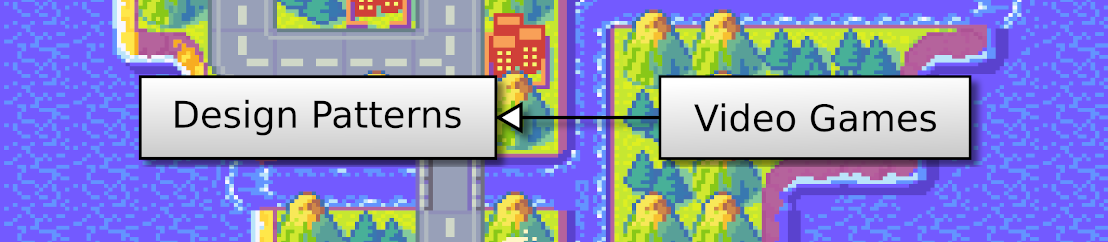
Programs in the previous post always lead to the same result. Here, I show you the basics of branching, or how to change the flow of a program depending on cases.
This post is part of the Discover Python and Patterns series
If we want to display a message when the player types a specific word, we can use the following syntax:
word = input("Enter the magic word: ")
if word == "please":
print("This is correct, you win!")
print("Thank you for playing this game!")
If you run this program, and type "please", then you see the message "This is correct, you win!" as well as the final message. If you don't, you only see the final message, "Thank you for playing this game!".
In this example, we use the if statement:
if <condition>:
<what to do if the condition is met>
<these lines are always executed>
The <condition>
is an expression that, if evaluated as True
, leads to the execution of the following block. If it evaluated as False
, then the following block is skipped.
In the example, the condition is word == "please"
and we can translate it into: is it true that word
equals "please"? Please note the double equal symbol "==": this is not the single equal symbol, which is the assignment. If you type word = "please"
, it means word takes the value "please", and it is evaluated as True
(because "please" is not None
). Throughout the series, I'll show you many cases of condition expressions.
To understand what the if
statement executes, you need to understand blocks in Python. In this language, indentation defines blocks: all lines with the same combination of space symbols before the expression are in the same block.
In the example, we have a single line print("This is correct, you win!")
with an indentation of four spaces. If we want to add a second line to this block, we must also start it with four spaces:
word = input("Enter the magic word: ")
if word == "please":
print("This is correct.")
print("You win!")
print("Thank you for playing this game!")
The second line can't begin with 3 or 5 spaces or a tab symbol with a width of 4 spaces. It must begin with the same combination of space symbol as the first line of the block. If we want to use, for instance, an indentation of 3 spaces, all lines of the block must begin with exactly 3 spaces. You can choose a different combination for each block.
In the previous example, if the player doesn't type the magic word, there is no specific display. We can also use the if statement to display a message for this case:
word = input("Enter the magic word: ")
if word == "please":
print("This is correct, you win!")
if not (word == "please"):
print("This is not correct, you lost :-(")
print("Thank you for playing this game!")
I added a new if
statement with the condition not (word == "please")
. The syntax not (<condition>)
means that we want to evaluate the opposite of a condition, in this example word == "please"
.
We can simplify this negation with the operator !=
:
if word != "please":
print("This is not correct, you lost :-(")
You can translate word != "please"
into: is it true what word is different from "please"?
The negation works fine, but we need to repeat the first condition. A more handy syntax is thanks to the else statement:
word = input("Enter the magic word: ")
if word == "please":
print("This is correct, you win!")
else:
print("This is not correct, you lost :-(")
print("Thank you for playing this game!")
This program has the same behavior as the previous one: if the player types the magic word, we display the winning message, otherwise the losing message.
Note that we must always put an if
statement before the else
statement. You can't use an else
statement without an if
or after a block that is not related to an if
statement.
In our example game, we can imagine more messages depending on what the player types. We can use the previous syntax to do so:
word = input("Enter the magic word: ")
if word == "please":
print("This is correct, you win!")
else:
if word == "mercy":
print("You must find the right one, you lost :-(")
else:
print("This is not correct, you lost :-(")
print("Thank you for playing this game!")
When the player types "mercy", we display a new message. Note the block of the else
statement:
if word == "mercy":
print("You must find the right one, you lost :-(")
else:
print("This is not correct, you lost :-(")
It contains four lines and two sub-blocks. The first sub-block is:
print("You must find the right one, you lost :-(")
And the second sub-block is:
print("This is not correct, you lost :-(")
We identify the blocks thanks to the indentation. For the block of the else
statement, each line starts with four spaces. Then, each sub-block also starts with four spaces, to which we added four more spaces. These sub-blocks must start with four spaces, as for every line of the else
block. Then, we can freely add any combination of spaces to create sub-blocks. In this example, and as for most of the programs in this series, I added four more spaces, but I could add 3 or 5 more spaces.
To help you understand indentation better, select the lines of the else
statement in Spyder:
Then, hit Shift+Tab or select the menu Edit / Unindent. You get the following result, where Spyder complains about the wrong indentation of the first line of the else
statement:
If you hit Tab or select the menu Edit / Indent, you come back to the initial state of the program.
You can play with these two operations, "Unindent" and "Indent" to see what happens when you create or delete new blocks, sub-blocks, sub-sub-blocks, etc.
The previous syntax works well but quickly becomes complex if we want to add more cases. We can get a better syntax with the elif
statement:
word = input("Enter the magic word: ")
if word == "please":
print("This is correct, you win!")
elif word == "mercy":
print("You must find the right one, you lost :-(")
else:
print("This is not correct, you lost :-(")
print("Thank you for playing this game!")
You can repeat as many elif
statements as you want after the if
statement. Then, you can add an else
statement if you need to, but this is not mandatory.
In this post, we see how to change the behavior of the program depending on the player, but we have to restart it to try again. In the next post, I'll show you how to use loops to repeat a block as much as necessary.