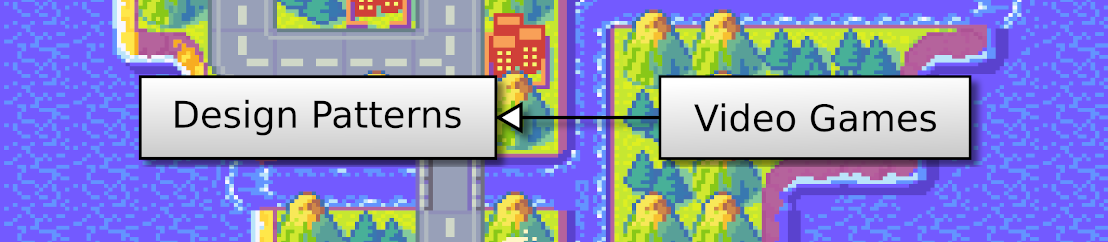
The previous game only allows one try: you have to restart it to propose another word. In this post, I introduce loops, and I use them to repeat the game until the player finds the magic word.
This post is part of the Discover Python and Patterns series
The while
statement allows to repeat a block until a condition is met:
while True:
word = input("Enter the magic word: ")
if word == "please":
print("This is correct, you win!")
else:
print("This is not correct, try again!")
print("Thank you for playing this game!")
The while
statement has the following syntax:
while [condition]:
[what to repeat]
[these lines are not repeated]
It repeats the sub-block as long as the condition evaluates as True
. This condition works in the same way as in the if
statement.
If you run this program, the game repeats forever, and the final message "Thank you..." is never displayed. To stop its execution in Spyder, close the console window. It is as expected since the condition in the while
statement is always True
.
To end the program when the player finds the magic word, we can use a variable that states if the game is over or not:
repeat = True
while repeat:
word = input("Enter the magic word: ")
if word == "please":
print("This is correct, you win!")
repeat = False
else:
print("This is not correct, try again!")
print("Thank you for playing this game!")
We initialize the variable repeat
as True
(line 1). Note that we can name this variable as we want, we don't have to name it repeat
, as long as the name is not already used. Then, we repeat as long as repeat
is True
(line 2). If the player finds the magic word (line 4), we print the winning message (line 5) and set repeat
to False
. In this case, the next time the while
condition is evaluated, it is False
, and the loop stops.
The previous approach does not stop the loop immediately. It means that we still execute the lines following repeat = False
, even if we want to stop the loop. We can get a better result with the break
statement:
while True:
word = input("Enter the magic word: ")
if word == "please":
print("This is correct, you win!")
break
else:
print("This is not correct, try again!")
print("Thank you for playing this game!")
When the break
statement is executed (line 5), the loop ends, and we go straight to line 8. Note that we no more need to introduce a variable to control the flow.
Another way to control loops is thanks to the continue
statement:
while True:
word = input("Enter the magic word: ")
if word != "please":
print("This is not correct, try again!")
continue
print("This is correct, you win!")
break
print("Thank you for playing this game!")
The continue
statement does not stop the loop; it goes to the beginning of the loop directly, as if the loop block was ending where continue
is. Note that the while
condition is evaluated before continuing the loop.
In the example, if the player doesn't type the magic word (line 3), we display a message (line 4), and we stop the current block (line 5) and go back to the beginning of the loop (line 1).
The break
and continue
statements can be in any sub-block of a loop, for instance, in an if
or else
block, except if there is a sub-loop. For instance, if there is a while
inside a while
, the break
statement stops the loop it is within, not the top one:
while True:
while True:
word = input("Enter the magic word: ")
if word == "please":
print("This is correct, you win!")
break
else:
print("This is not correct, try again!")
word = input("Type 'again' to play again: ")
if word != "again":
break
print("Thank you for playing this game!")
This program runs the game as before in lines 2-8. It works the same, being in a loop does not change its behavior. The break
statement in line 6 stops this loop.
The main loop in lines 1-11 repeats the game if the player types "again". The break
statement in line 11 stops the main loop.
If we want the sub-loop in lines 2-8 to stop the main loop, we have to use a variable:
repeat = True
while repeat:
while True:
word = input("Enter the magic word: ")
if word == "please":
print("This is correct, you win!")
break
elif word == "quit":
repeat = False
break
else:
print("This is not correct, try again!")
print("Thank you for playing this game!")
We initialize a repeat
variable to True
. As long as it is True
, the main loop continues (line 2). If the player types "quit" (line 8), we set repeat
to False
(line 9), and stop the sub-loop (line 10). Then, since repeat
is False
, the main loop stops and we display the final message (line 13).
Loops allow us to repeat an action or a process as much as required. I used it to create a small game where you have to guess a word. In the next post, I'll introduce data types and show you how to do the same with numbers.